Initiate an Immediate Transfer
Flow
The Immediate payment flow using the Fintecture Connect webview has been simplified into the following steps and illustrated using the payment flow below:
- Request a PIS dedicated access token
- Create a Payment Session and redirect the payer (i.e. PSU) to the returned Connect URL
- Verify the payment on callback such that the payment status and order ID matches
- Listen to Webhook to intercept payments status change events as a redundant channel to the redirect callback
🚧 Important
Signature, digest, date and x-request-id headers are optional in SANDBOX environment but mandatory when calling the PRODUCTION one.
B2C Recipe B2B Recipe Postman Diagram💡 Note
Step 4 is identified as optional but as the payment method relies mostly on a redirection authentication model, it is important to use a redundant payment notification channel in case the redirection fails. Some implementations use webhooks as the main payment notification channel and the redirection simply used to display the resulting payment result.
Payment statuses
Below are detailed the payment statuses encountered in the case of an immediate payment.
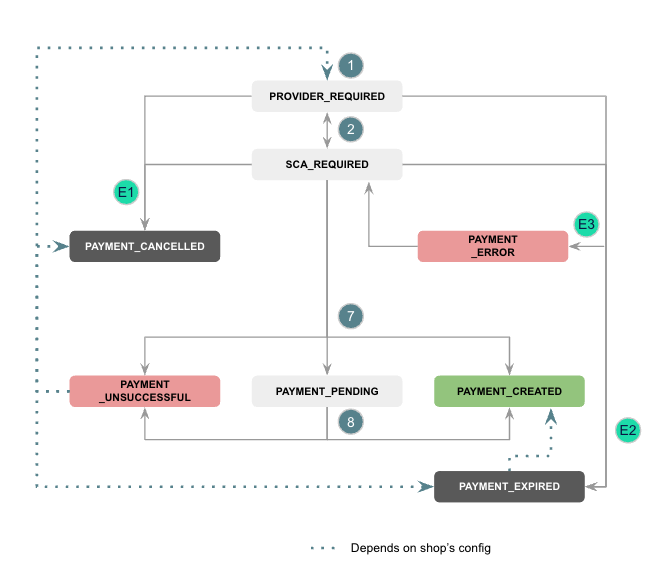
💡 Note
To ease reproducing some of your scenarios, here are some tips to reach the different statuses in SANDBOX environment.
💡 Note
Do not hesitate to consult all of the payment statuses for more information.
1. Request an Access Token
The first step is to authenticate your application with the Fintecture API Gateway and this is done using the /access token API, as illustrated.
To use the API, you must first create a basic token. To do so, encode the following string using a base64 encoder:
`basic_token` = base64(`app_id`:`app_secret`)
Include your app_id
and basic_token
the the following request:
POST /oauth/accesstoken HTTP/1.1
Authorization: Basic [basic_token]
Accept: application/json
Content-Type: application/x-www-form-urlencoded
grant_type=client_credentials&app_id=[app_id]&scope=PIS
The expected response is the following:
{
"token_type": "Bearer",
"access_token": "eyJhbGciOiJub25lIn0.eyJleHAiOjE1MTQwODA0MjQsI...",
"expires_in": 3600
}
Store the access_token
for the next step.
2. Create a Payment Session
The second step is to create a Payment Session to receive the connect URL which will enable to user to complete his payment flow.
Simply POST the illustrated request including all the necessary headers, body and query string. For more information on each field, see the /connect API
POST /pis/v2/connect?redirect_uri=[redirect_uri]&state=[state] HTTP/1.1
Authorization: Bearer [access_token]
Signature: [signature]
Digest: [digest]
Date: [date]
x-request-id: [request_id]
Accept: application/json
Content-Type: application/json
{
"meta": {
"psu_name" : "Julien Lefebre",
"psu_company": "My Business SARL"
"psu_email" : "[email protected]",
"psu_phone" : "09743593535",
"psu_incorporation": "123456789",
"psu_address": {
"street": "rue Marie Stuart",
"number": "2",
"complement": "2nd floor",
"zip": "75002",
"city": "PARIS",
"country": "FR"
}
},
"data": {
"type" : "payments",
"attributes" : {
"amount" : "273",
"currency": "EUR",
"communication" : "B34970692"
}
}
}
The expected response is the following
{
"meta": {
"session_id": "44f00841780445d4981be9ea2f8aafae",
"url": "https://connect-v2.fintecture.com/v2/00547d75-243e-48ce-9b0c-12136c076a8a"
}
}
The response of a successful request is the Connect URL and the corresponding payment session_id which will enable you to follow the transaction using the /payments/[session_id] API
After redirecting the PSU to the Connect URL, they will be able to select their bank and initiate the payment from their bank's portal. Following the payment initiation, they will be redirected back to your redirect_uri with the following query string parameters:
session_id
: the payment session_idstatus
: the status of the payment (see status section for more information on the status)provider
: the bank code which the PSU connected tocustomer_id
: the identifier of the payment userstate
: the state parameter which you provided during the PIS Connect URL
3. Fetch the Payment Status
The payment validation is verifying that the payment has either been successful or not.
Start by considering the returned parameters from the callback. The redirection url is composed of the following parameters:
redirect_uri?session_id=session_id&status=status&provider=provider&customer_id=customer_id&state=state
Using the callback query string parameter session_id, you can call the API at the /payments/[session_id] endpoint. The returned values from the /payments/[session_id] endpoint are the actual payment values you can consider.
On your end, retrieve the order reference using the callback state query parameter which you stored locally. Reconcile the order reference and only then can you validate the payment and consider the payment status from the returned payload.
Reconciliation tipThe merchant order reference should be sent in the communication field of the payment as to be able to validate the payment on callback and enable refund.
GET pis/v2/payments/[session_id] HTTP/1.1
Accept: application/json
Authorization: Bearer [access_token]
Signature: [signature]
Date: [date]
X-Request-Id: [x-request-id]
The expected response will be
{
"meta": {
"session_id": "44f00841780445d4981be9ea2f8aafae",
"status": "payment_created",
"code": 200,
"provider": "cmcifr2a",
"is_accepted": true,
"has_settlement_completed": true,
"type": "PayByBank",
"initial_type": "PayByBank",
"customer_id": "3621eacaccd04fa772638dec70da323c"
},
"data": {
"id": "44f00841780445d4981be9ea2f8aafae",
"type": "payments",
"attributes": {
"amount": "273",
"currency": "EUR",
"communication": "B34970692",
"end_to_end_id": "44f00841780445d4981be9ea2f8aafae",
"execution_date": "2021-02-28",
"payment_scheme": "SEPA",
"transfer_state": "completed",
"bank_account_id": "ee6f357e-e49c-4ff2-954f-b92f237cc800",
"account_type": "external"
}
}
}
4. Webhooks
Webhooks enables you to be notified of an event such as a payment status change.
In the context of a payment model based on redirection, it is important to use a redundant payment notification channel in case the redirection fails. Some implementations uses webhooks as the main payment notification channel and the redirection simply displays the resulting payment result.
You can add webhooks to your application using the Console. The configuration of a webhook requires the following three parameters:
url
: The URL to which the event message will be sentoffset
: The delay in minutes after which the event message will be sent once an event occursevent
: The list of events to which you want to subscribe the webhook
The webhook is a x-www-form-urlencoded POST request which is signed using your public key. Verify the signature using your private key, and only then process the order based on the result of the payment.
Exponential Time IncrementWebhook will retry 10x with an exponential time increment if your sever doesn't respond with a HTTP code in the 2XX range.
The HTTP request made by our servers to your endpoint will be similar to the following:
POST /webhook HTTP/1.1
Host: mywebsite.com
Signature: keyId="2dfdcf57-5b2f-4309-846f-913d0b2802cf",algorithm="rsa-sha256",headers="date digest x-request-id",signature="h0V0SUbjRhLEP/MiYo0Mgs1N17EuCEmKyQrDjxysc7iSiFXTjvY6qVEoaiRkzB8ZI0J39gGwOtTXN9CJPVRbhEHhi9Z9rQvM33FkygXvvx8BwM76fSTQ2/BSZWx04CjbPv/XUVusnkKVr3W6p+Vn073hAuJn1nKCvDOyl+QnDtstkzT+UacVzDA9L9nyPbbaPQHJobaZuG8TjhnI+Y0PZxneke6OU6fcdPT0uwkEamDOOExcMryHIX1iH5iiPMvLoVA8acqvvMSDYar0rlEQ2J1M4dcowWT8FxLo6C8uqvJIaBYm7Ze0RNJOwY0UBImCVDIuQLJuBjPwjQT5GjTQlg==
Digest: SHA-256=wOtTXN9CJPVRbhEHhi9Z9rQvM33FkygXvvx8BwM76fS
Date: Mon, 08 Jun 2020 23:11:23 GMT
X-Request-ID: 88c414df-6895-48db-8ef3-1fd1ce4272c6
Content-Type: application/x-www-form-urlencoded
session_id=b2bca2bcd3b64a32a7da0766df59a7d2&status=payment_created&customer_id=1ef74051a77673de120820fb370dc382&provider=provider&state=thisisastate
Updated 11 days ago